A guide for creating respec tests
Having completed a boot camp and now rebooting my learning with exercism I wanted to start creating my own tests for some “puzzle” ideas I want to develop. On the advise of a senior developer I created a tennis game and use rspec test to help drive this game development. Funny enough this is also a very topical sport to target with the Djokovic saga going on too..
Setting up a rspec test environment:
Create folders & implementation file
Open a terminal
mkdir <game_name>
mkdir <game_name>/lib
cd <game_name>/lib
touch game.rb
Creating the rspec environment
back in terminal
cd ..
code . #opens your project
bundle init
this will create a Gemfile
- open Gemfile and modify
- remove gem “rails” and change to gem “rspec”
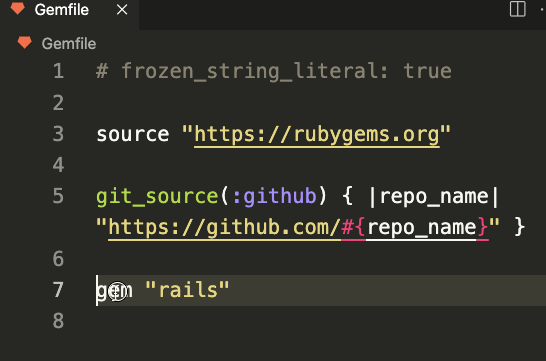
bundle
rspec --init
touch spec/<game>_spec.rb
Now you have created a rspec test folder and test file
<game_name>/spec/
Creating a rspec test:
Open the <game_name>/spec/
RSpec.describe <Game> do
it "what does the implemntation" do
expect(method).to eq "expected outcome"
end
end
Two test examples for my tennis game when you want the scores to be Test 1: “Deuce” or Test 2: “Love all”
RSpec.describe Tennis do
# Test 1
it 'updates the score when server and receiver both win three points each' do
tennis = Tennis.new
# pending
3.times{tennis.server_wins_point}
3.times{tennis.receiver_wins_point}
expect(tennis.score).to eq 'Deuce'
end
# Test 2
it 'reports the starting score ' do
tennis = Tennis.new
expect(tennis.score).to eq 'love all'
end
end
Running a rspec test:
back in terminal to run the rspec test:
rspec <game>_spec.rb
Passing and Failed Tests
Now that we have a test we can use the tests to drive our developer enironment we want to turn RED into GREEN
![]() |
![]() |
---|---|
Green Passed Tests |
RED Failed test need some more work |
Extra nice to know about a rspec tests:
- eq means an equality match but there are others you can use in the rspec test .
- note: method may need to be a class.method eg Tennis.score
- use pending to skip a given test